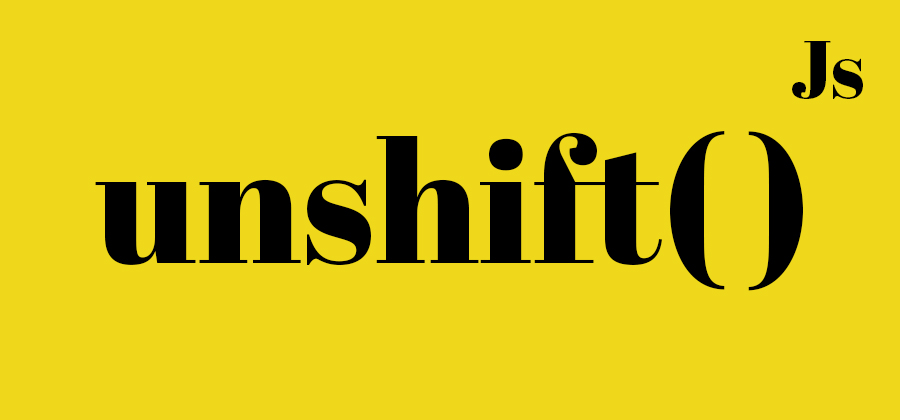
In JavaScript, the unshift() method is used to add one or more elements to the beginning of an array. This allows you to insert items at the start, which shifts all existing elements one position forward.
Here’s how the unshift() method works and how to use it in your code.
What Does unshift() Do?
The unshift() method in JavaScript:
- Adds one or more elements to the start of an array.
- Shifts all existing elements to the right.
- Returns the new length of the array.
Example: Using unshift() to Add an Element to the Beginning
Let’s say we have an array of fruits:
var fruits = ['apple', 'banana', 'orange'];
If we want to add 'kiwi' to the beginning of this array, we can use the unshift() method like this:
fruits.unshift('kiwi'); console.log(fruits); // Output: ['kiwi', 'apple', 'banana', 'orange']
Explanation
- Step 1: Declare an array named fruits with the values ['apple', 'banana', 'orange'].
- Step 2: Use fruits.unshift('kiwi') to add 'kiwi' at the beginning of the array.
- Step 3: Log the updated array to see the new values: ['kiwi', 'apple', 'banana', 'orange'].
When to Use unshift()?
The unshift() method is perfect for:
- Adding elements to the beginning of an array.
- Working with lists where the order matters, and you want the new item to come first.
By using unshift(), you can easily add items at the start of an array, making it a useful tool for array manipulation in JavaScript.
No comments:
Post a Comment