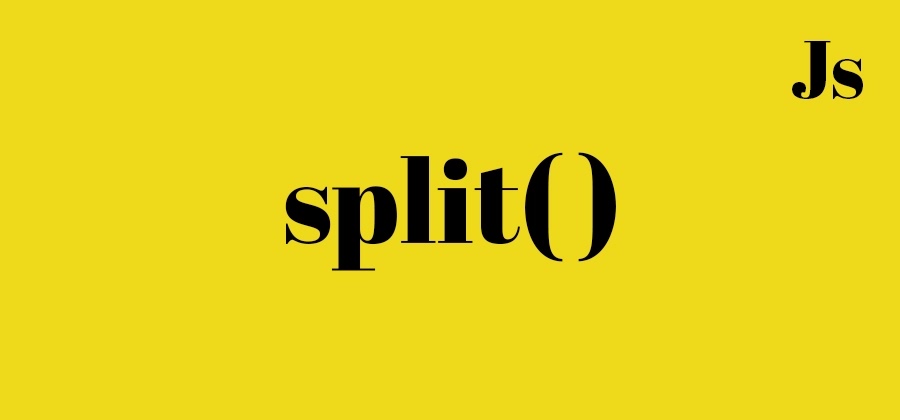
The split() method in JavaScript allows you to transform a string into an array by dividing it at specified characters. This is useful when you want to separate a sentence into individual words, characters, or parts based on a delimiter, such as a space or comma.
What Does split() Do?
The split() method in JavaScript:
- Divides a string into an array of substrings.
- Uses a specified separator (like a space, comma, or any other character).
- Returns an array of the split elements.
Example: Using split() to Convert a String into an Array
Let’s look at an example where we split a sentence into individual words:
var stringOne = "My name is Gokul"; var stringOutput = stringOne.split(" "); console.log(stringOutput); // Output: ["My", "name", "is", "Gokul"]
Explanation
Step 1:Declare a variable stringOne with the value `"My name is Gokul"`.
Step 2:Use `stringOne.split(" ")` to split the sentence into an array of words. Here, " " (a single space) is specified as the separator.
Step 3:Log the output to see the result: `["My", "name", "is", "Gokul"]`.
Additional Options with split()
If you omit the separator or provide an empty string (""), split() will divide the string into individual characters. For example:
var stringOne = "Gokul"; var characterOutput = stringOne.split(""); console.log(characterOutput); // Output: ["G", "o", "k", "u", "l"]
When to Use split()?
The split() method is ideal when:
- You need to break down a string into individual words, characters, or parts.
- You want to create an array from a sentence or phrase for further manipulation.
By using split(), you can effectively convert strings to arrays in JavaScript, allowing you to work with each part of the text independently.
No comments:
Post a Comment